Project Description
The project is an automated hydroponic plant growth system for small plants which uses a pump, reservoir, tubing to periodically spray water particles with nutrients onto the roots of the plant. The device is made up of ESP32 CPU and various sensors to record plant growth conditions. The project includes a full implementation of a user interface that incorporates a frontend, backend, and database to ensure that the user can interact with the CPU as well as view growth conditions.
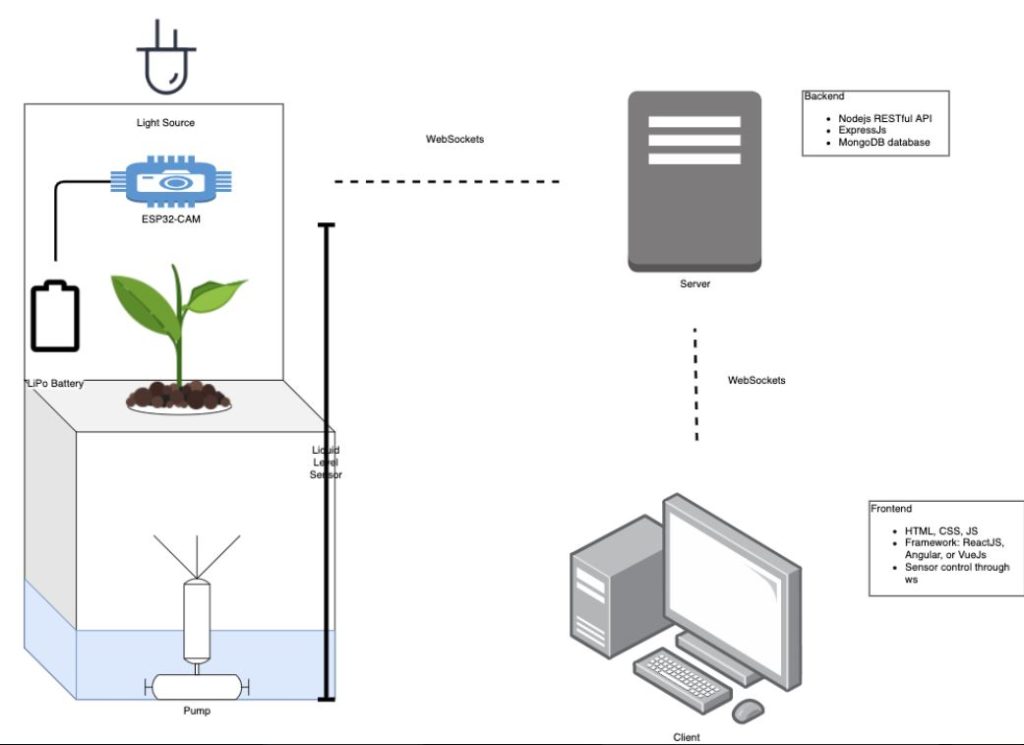
Software
Full code available to view on GitHub.
Home Page
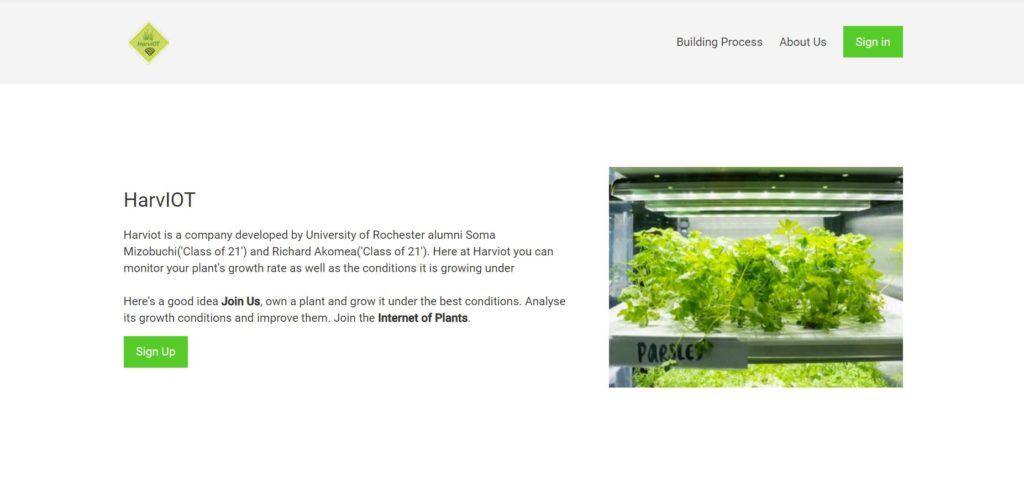
Data Models
//Sign-In data model
class SignInData{
private email:string;
private password:string;
constructor(email:string, password:string){
this.email = email;
this.password = password;
}
getEmail():string{
return this.email;
}
getPassword():string{
return this.password;
}
}
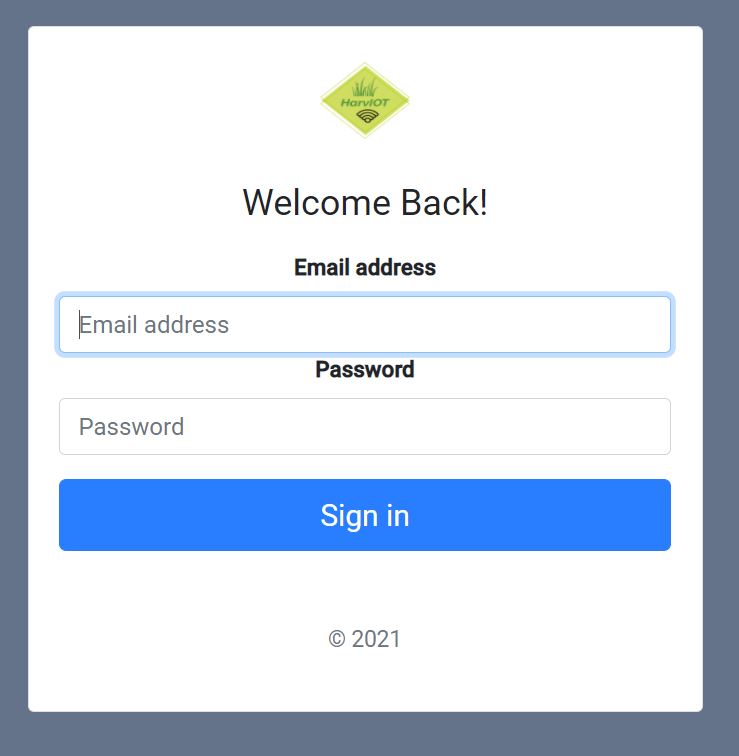
//Sign up data model
class RegisterData{
private firstName:string;
private lastName:string;
private email:string;
private password:string;
constructor(firstName:string, lastName:string, email:string, password:string){
this.firstName = firstName;
this.lastName = lastName;;
this.email = email;
this.password = password;
}
getFirstName():string{
return this.firstName;
}
getLastName():string{
return this.lastName;
}
getEmail():string{
return this.email;
}
getPassword():string{
return this.password;
}
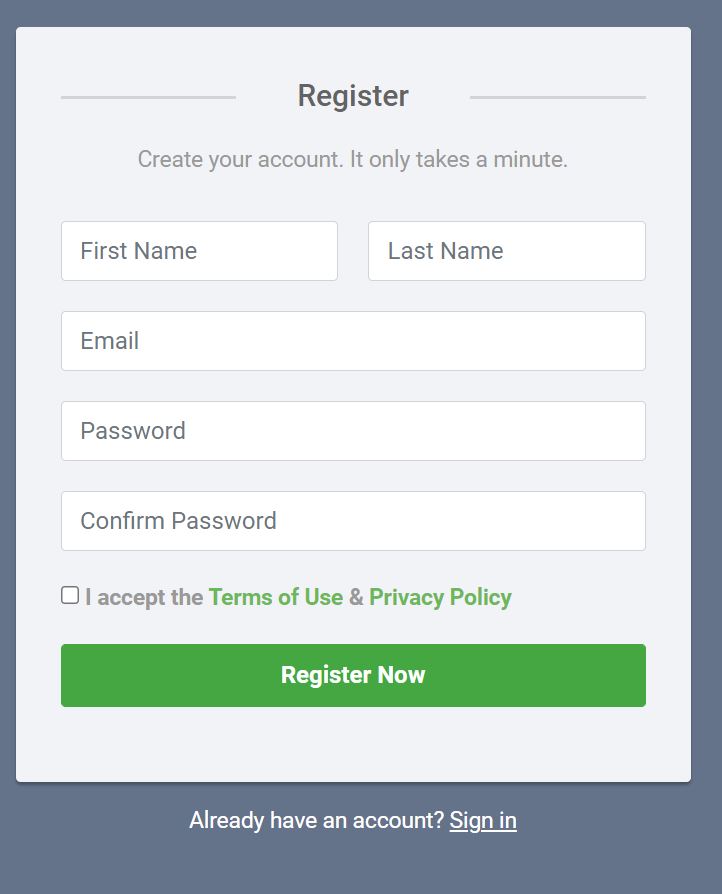
Upon login:
//when login form is submitted
onSubmit(signInForm: NgForm){
//if data doesn't match in database
const signInData = new SignInData(signInForm.value.email, signInForm.value.password);
if(!this.authenticationService.checkCredentials(signInData)){
this.isFormInvalid = false;
this.areCredentialsInvalid = true;
return;
}
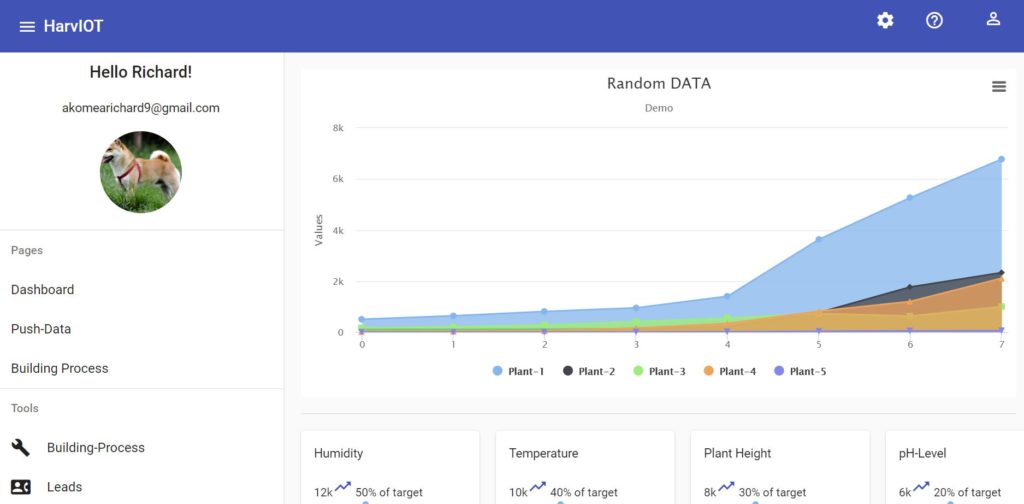
<!-- USER DASHBORD ... TOGGLES BETWEEN DATA DISPLAYS/SEND -->
<div *ngIf="authenticationService.returnAuth()">
<app-header (toggleSideBarForMe)="sideBarToggler()"></app-header>
<mat-drawer-container>
<mat-drawer mode="side" [opened]="sideBarOpen">
<app-sidebar></app-sidebar>
</mat-drawer>
<mat-drawer-content [ngSwitch]="message">
<app-dashboard *ngSwitchDefault></app-dashboard>
<app-physical *ngSwitchCase="'phys'"></app-physical>
<app-dashboard *ngSwitchCase="'dash'"></app-dashboard>
</mat-drawer-content>
</mat-drawer-container>
<app-footer></app-footer>
</div>
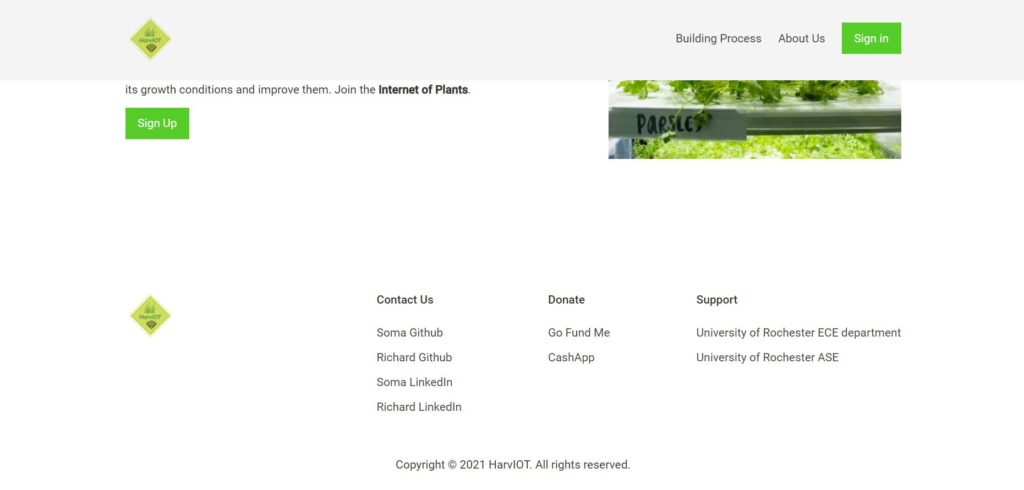
Hardware
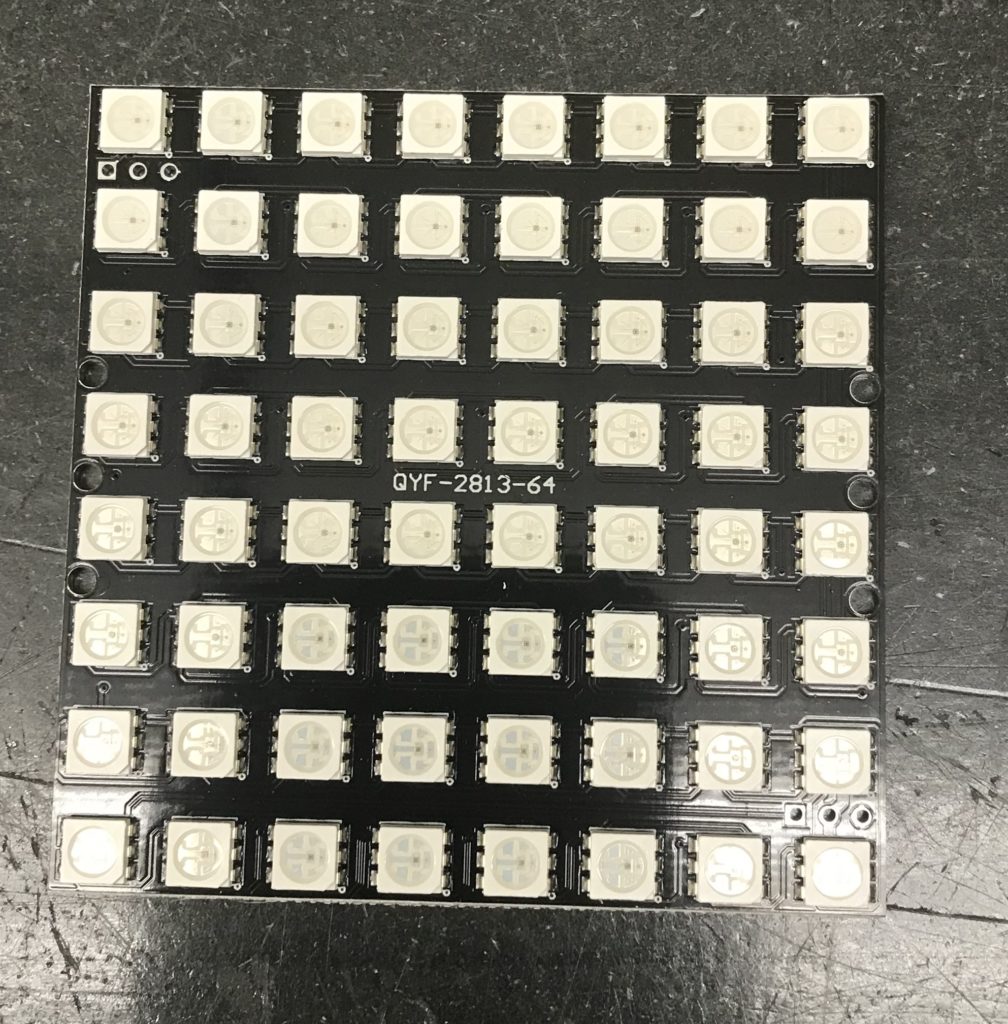


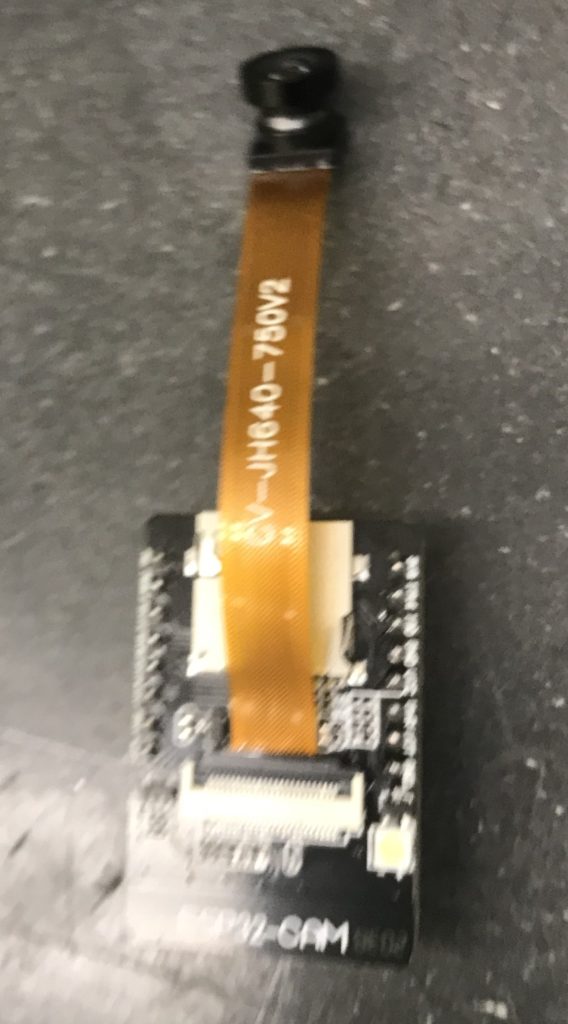
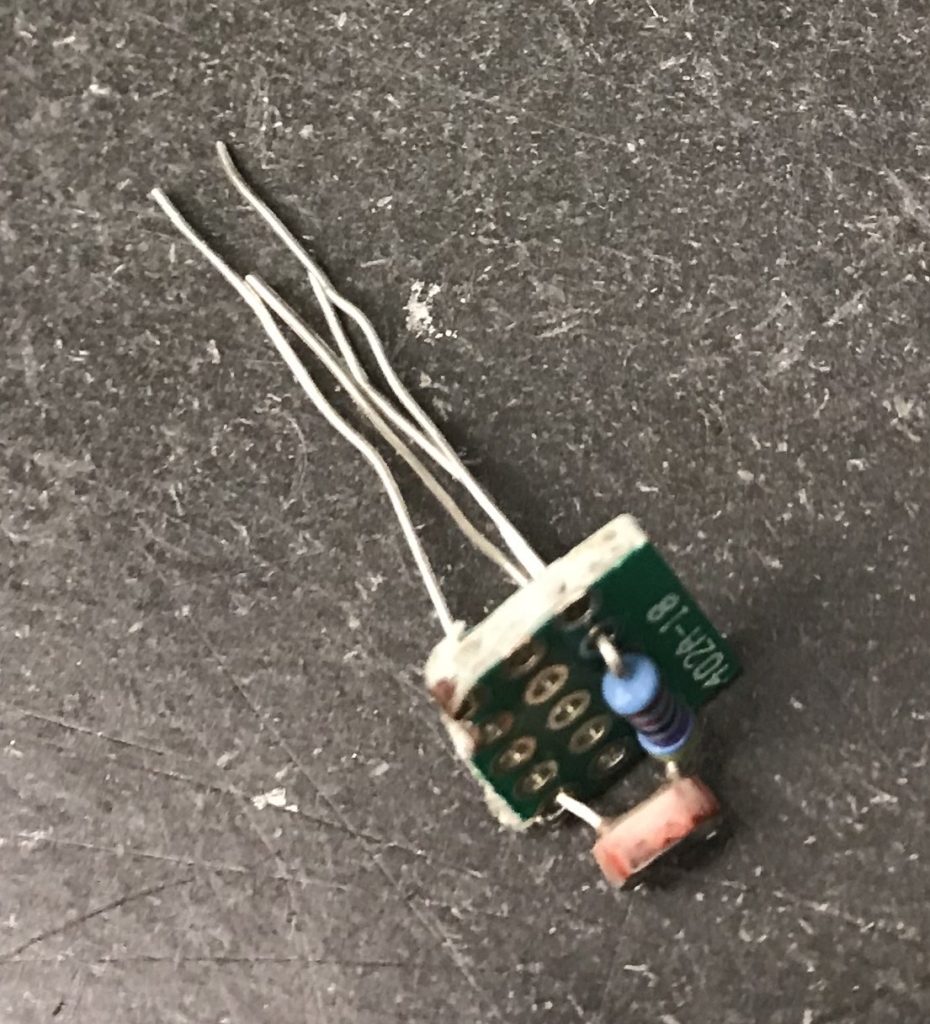

Demo Data Dump
Below are the first five(objects) representing plant data from our database. This information is available to view (in full) at the response.json file in the assets folder of the GitHub repository.
Temperature | Humidity | Ambient Light | Date/Time |
24.52553 | 36.98019 | 6864 | 2021-04-29T20:19:53 |
24.5148 | 37.00308 | 6848 | 2021-04-29T20:19:50 |
24.53625 | 36.99545 | 6848 | 2021-04-29T20:19:47 |
24.50407 | 37.00308 | 6880 | 2021-04-29T20:19:44 |
24.52553 | 36.97256 | 6816 | 2021-04-29T20:19:41 |
Final Product/Demo
Observations / Points to improve in the future
- CPU/System Cooling: We observed that the board got really hot when the system was left running for a long time. The system overheated quite a few times resulting in us replacing multiple ESP32 chips.
- Product Aesthetic: Our product wasn’t put together in the most elegant way and has space for improvement.